In this blog, we will show you the way to create a custom logger to log your own messages into it.
Step 1: Create a logger class
Firstly, create a logger class:
app/code/Magenest/CustomLog/Logger/Logger.php
<?php namespace Magenest\CustomLog\Logger; class Logger extends \Monolog\Logger { }
app/code/Magenest/CustomLog/Logger/Handler.php
<?php namespace Magenest\CustomLog\Logger; use Monolog\Logger; class Handler extends \Magento\Framework\Logger\Handler\Base { /** * Logging level * @var int */ protected $loggerType = Logger::NOTICE; /** * File name * @var string */ protected $fileName = '/var/log/magenest_custom_log.log'; }
The file name /var/log/magenest_custom_log.log
is a relative path to the magento root folder, it means your log will be stored under <magento_root>/var/log/magenest_custom_log.log
The variable loggerType
define the log level can be applied.
Monolog/Logger
has the following consts:
- DEBUG = 100
- INFO = 200
- NOTICE = 250
- WARNING = 300
- ERROR = 400
- CRITICAL = 500
- ALERT = 550
- EMERGENCY = 600
For example, loggerType = NOTICE. It means the logger level will be from “NOTICE” and above such as “WARNING”, “ERROR”, “CRITICAL”, ... And can not log the level “DEBUG” and “INFO”.
Step 2: Declare the logger
The next step is to declare the logger in di.xml.
app/code/Magenest/CustomLog/etc/di.xml
<?xml version="1.0"?> <config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="../../../../../lib/internal/Magento/Framework/ObjectManager/etc/config.xsd"> <type name="Magenest\CustomLog\Logger\Handler"> <arguments> <argument name="filesystem" xsi:type="object">Magento\Framework\Filesystem\Driver\File</argument> </arguments> </type> <type name="Magenest\CustomLog\Logger\Logger"> <arguments> <argument name="name" xsi:type="string">Magenest_Custom_Log</argument> <argument name="handlers" xsi:type="array"> <item name="system" xsi:type="object">Magenest\CustomLog\Logger\Handler</item> </argument> </arguments> </type> </config>
You have defined the handler with a unique name. The class Magenest\CustomLog\Logger\Logger
will be handled by the handler Magenest\CustomLog\Logger\Handler
That’s it, your logger is ready to use. To test our logger, we will create a controller as the following:
app/code/Magenest/CustomLog/Controller/Index/Index.php
<?php namespace Magenest\CustomLog\Controller\Index; use Magenest\CustomLog\Logger\Logger; use Magento\Framework\App\Action\Action; use Magento\Framework\App\Action\Context; use Magento\Framework\View\Result\PageFactory; class Index extends Action { /** * Index action * * @return $this */ /** @var PageFactory */ protected $resultPageFactory; /** * @var Logger */ protected $logger; public function __construct( Context $context, PageFactory $resultPageFactory, Logger $logger ){ $this->resultPageFactory = $resultPageFactory; $this->logger = $logger; parent::__construct($context); } public function execute() { $this->logger->error('Log Error'); $this->logger->emergency('Log Emergency'); $this->logger->info('Log Info'); $this->logger->debug('Log Debug'); $resultPage = $this->resultPageFactory->create(); return $resultPage; } }
Now you can find the log in var/log/magenest_custom_log.log
file

As you can see, our logger can only print the log with the level from “NOTICE” and above, the lower level will not be printed.
Hope this guide is useful for you to create custom logger in Magento 2
Thank you for reading our blog and see you in the upcoming articles.
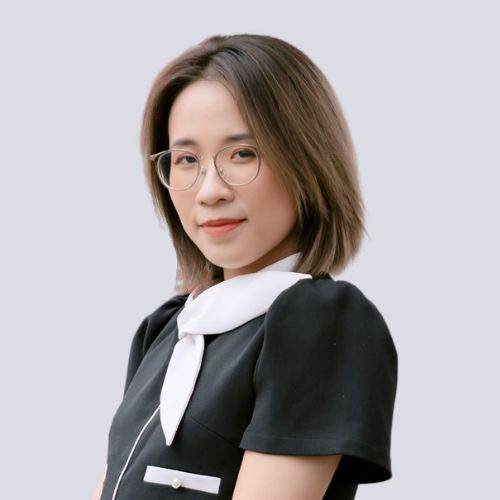