Overview
According to recent market surveys, about 12% of all online retail websites were developed on the Magento platform. As of October 2019, Builtwith.com's statistics, there are more than 250,000 e-commerce websites running on the platform.
Even though Magento is a highly secure CMS compared to others, but still, it's still necessary to make sure that your Magento store is secure against all the risks of hacking attacks. Currently, Magento has provided updated patches that contain many security fixes and functionality.
Altering the information could possibly protect it from unauthorized access, and as a result, the only authorized receiver can understand it. This is called encrypting and decrypting data, which is supported by the Magento framework out-of-the-box.
Implementation
Magento 2’s encryption and decryption use Magento\Framework\Encryption\EncryptorInterface
, which is implemented by Magento\Framework\Encryption\Encryptor
class.
Coding example:
protected $encryptor; public function __construct( Context $context, \Magento\Framework\Encryption\EncryptorInterface $encryptor ) { $this->encryptor = $encryptor; parent::__construct($context); } /** * Encrypt data * * @param string $data * @return string */ public function encrypt() { $password = "magenest"; $encrypt = $this->encryptor->encrypt($password); return $encrypt } /** * Decrypt value * * @param string $value * @return string */ public function decrypt($value) { return $this->encryptor->decrypt($value); }
First, we need to define EncryptorInterface class
use Magento\Framework\Encryption\EncryptorInterface;
After that, we need to declare one variable inside our class.
protected $encryptor;
Next up, we bind the variable to EncryptorInterface inside the class’s constructor:
$this->encryptor = $encryptor;
Finally, call the encrypt function to encrypt the given information.
- $password is converted to a form that cannot be understood by the user before saving it to the database.
- After using the original data encryption function "magenest" will be encrypted into a similar string of type "0:3:57b1KnIoivsjoETztgLcO39loK87yHi/ItNANgjH2idUh2Gv" this will ensure that your password will be kept the highest security.
Decryption:
- We use the function decrypt() of the EncryptorInterface class.
- This returns the original $password string
These simple methods are widely used in core and custom Magento 2 modules.
We hope these simple steps can assist you in Encryption and Decryption in Magento 2. If you have any questions, please put them in the comment.
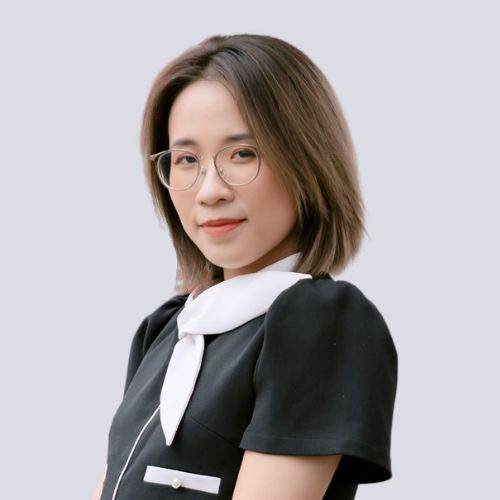
Good day
Above Magento 2 encrypt and decrypt is good.
But I have one question can we encrypt and decrypt with our custom key.
I tried with many search but couldn't found any links that can encrypt and decrypt with custom keys
let me know if know something about this.
Appriaciate your help
Thanks