What is API in Magento?
Application Programming Interface (API) allows access to the data from an application. Magento by default provides all the APIs such as Product API, Order API, Customer data API, etc. But if we add any custom fields or want to manage custom data, we need to create new API.
As per the REST (REpresentational “State” Transfer) architecture, the server does not store any state about the client session on the server side. This restriction is called Statelessness. Each request from the client to server must contain all of the information necessary to understand the request, and cannot take advantage of any stored context on the server.
Let’s take a look at how we create a new REST API.
API Configuration
Create file webapi.xml in app/code/Magenest/Customapi/etc/
webapi.xml is the configuration file for all the API of a module.
<?xml version="1.0"?> <routes xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Webapi:etc/webapi.xsd"> <route url="/V1/customer/me" method="POST"> <service class="Magenest\Customapi\Api\CustomerGreetingInterface" method="sayHello"/> <resources> <resource ref="self"/> </resources> </route> </routes>
- Attribute URL: the route for the web service.
- Attribute method: is one of GET, PUT, POST or DELETE.
- Service Class: the interface class that handles the API and method is where the logic happens when the API is called.
- Resource: defines which users can access this API. Possible options are self, anonymous or Magento resource like Magento_Catalog::products or Magento_Customer::group, etc.
Then, we declare the implementation for the API interface in app/code/Magenest/Customapi/etc/di.xml
<?xml version="1.0"?> <config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="../../../../../lib/internal/Magento/Framework/ObjectManager/etc/config.xsd"> <preference for="Magenest\Customapi\Api\CustomerGreetingInterface" type="Magenest\Customapi\Model\ResourceModel\CustomerGreeting"/> </config>
Interface and ResourceModel
Create CustomerGreetingInterFace.php in app/code/Magenest/Customapi/Api/ and add declare the sayHello function.
<?php namespace Magenest\Customapi\Api; interface CustomerGreetingInterface { /** * Get customer's name by Customer ID and return greeting message. * * @api * @param int $customerId * @return \Magento\Customer\Api\Data\CustomerInterface * @throws \Magento\Framework\Exception\NoSuchEntityException If customer with the specified ID does not exist. * @throws \Magento\Framework\Exception\LocalizedException */ public function sayHello($customerId); }
The method needs to match with the one you have declared in webapi.xml
Then, define the Model in app/code/Magenest/Customapi/Model/CustomerGreeting.php.
Concrete class of the method must be defined and it should give the definition of all the API methods and should inherit the doc-block.
<?php namespace Magenest\Customapi\Model; use Magenest\Customapi\Api\CustomerGreetingInterface; use Magento\Customer\Model\CustomerRegistry; class CustomerGreeting implements CustomerGreetingInterface { /** * @var CustomerRegistry */ protected $customerRegistry; public function __construct(CustomerRegistry $customerRegistry) { $this->customerRegistry = $customerRegistry; } /** * Get customer's name by Customer ID and return greeting message. * * @api * @param int $customerId * @return \Magento\Customer\Api\Data\CustomerInterface * @throws \Magento\Framework\Exception\NoSuchEntityException If customer with the specified ID does not exist. * @throws \Magento\Framework\Exception\LocalizedException */ public function sayHello($customerId) { $customerModel = $this->customerRegistry->retrieve($customerId); $name = $customerModel->getDataModel()->getFirstname(); return "Hello " .$name; } }
This Model must implement the Interface that was declared previously in di.xml file. In this Model, as you can see, we create functions that will be executed by API method (In here, that is function sayHello).
And note that this function requires a parameter, so we need to pass the correct parameter for it to work.
Note:
- All the methods in Interface and Model should have a doc-block.
- If your method expects parameters than all the parameters must be defined in the doc-block as @params <type> <param> <description>
- Return type of the method must be defined as @return <type> <description>
Test
I create a new Customer with the first name is Karl.
To test the API, we use Postman. (https://www.getpostman.com/)
Enter the URL: Base_Url/rest/V1/customer/me
Method: POST
Body: {“customerId” : 1}
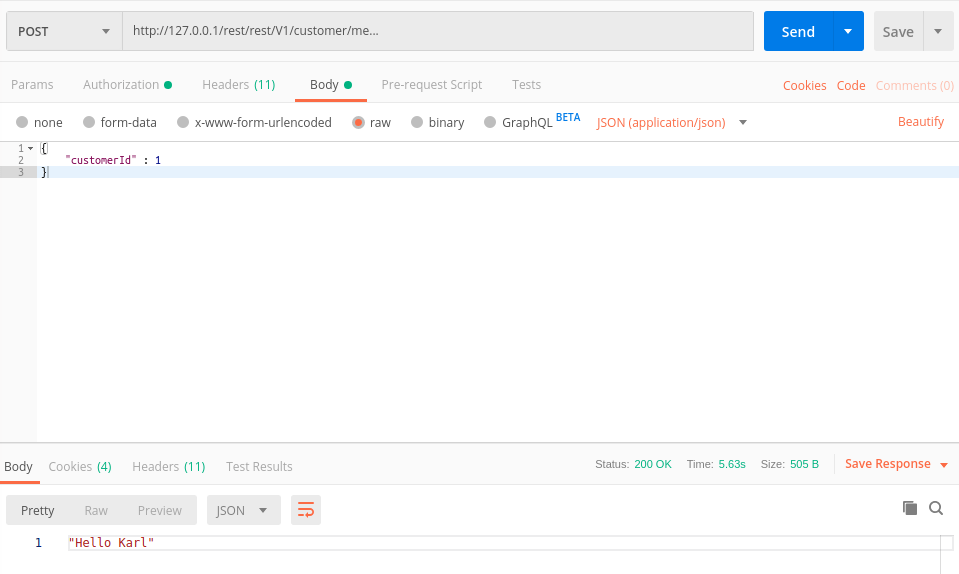
And the response "Hello Karl" is the expected output.
More specific details about Magento 2 REST APIs can be found here: https://devdocs.magento.com/guides/v2.3/get-started/bk-get-started-api.html
I hope you found this tutorial useful. Happy coding!
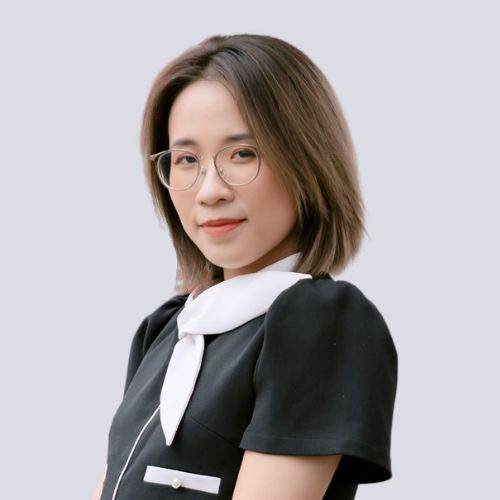
"message": "Request does not match any route."
}