By Magento default, Comment History of order is a feature that helps us add annotations to that order by adding it manually. However, you can add comments to the order programmatically.
This example will show you how to programmatically add comments to Order in Magento 2.
<?php namespace Magenest\Blogs\Model\Order; use Magento\Framework\Exception\NoSuchEntityException; use Magento\Sales\Api\OrderRepositoryInterface; use Magento\Sales\Model\Order; use Magento\Sales\Model\Order\Status\HistoryFactory; use Psr\Log\LoggerInterface; class Comment { /** * @var LoggerInterface */ protected $logger; /** * @var OrderRepositoryInterface */ protected $orderRepository; /** * @var HistoryFactory */ protected $orderHistoryFactory; public function __construct( OrderRepositoryInterface $orderRepository, HistoryFactory $orderHistoryFactory, LoggerInterface $logger ) { $this->orderRepository = $orderRepository; $this->orderHistoryFactory = $orderHistoryFactory; $this->logger = $logger; } public function addCommentToOrder() { $orderId = 6; // Update order with the id = 6 $comment = 'Delivery on way'; // Your comment content $status = \Magento\Sales\Model\Order::STATE_PROCESSING; // Change order status try { $order = $this->orderRepository->get($orderId); if ($order->canComment()) { $history = $this->orderHistoryFactory->create() ->setStatus(!empty($status) ? $status : $order->getStatus()) // Update status when passing $comment parameter ->setEntityName(\Magento\Sales\Model\Order::ENTITY) // Set the entity name for order ->setComment( __('Comment: %1.', $comment) ); // Set your comment $history->setIsCustomerNotified(true)// Enable Notify your customers via email ->setIsVisibleOnFront(true);// Enable order comment visible on sales order details $order->addStatusHistory($history); // Add your comment to order $this->orderRepository->save($order); } } catch (NoSuchEntityException $exception) { $this->logger->error($exception->getMessage()); } return $this; } }
After running the above code, here is the output:
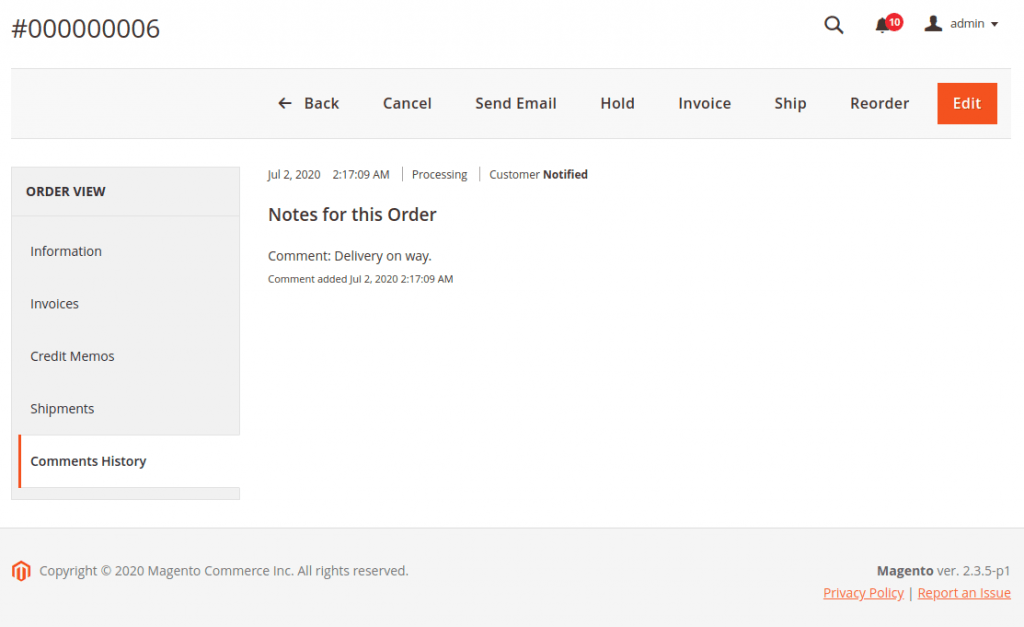
In this article, you can easily add comments to orders programmatically in Magento 2. Besides, you can also change the status of the order by passing an additional parameter in the method.
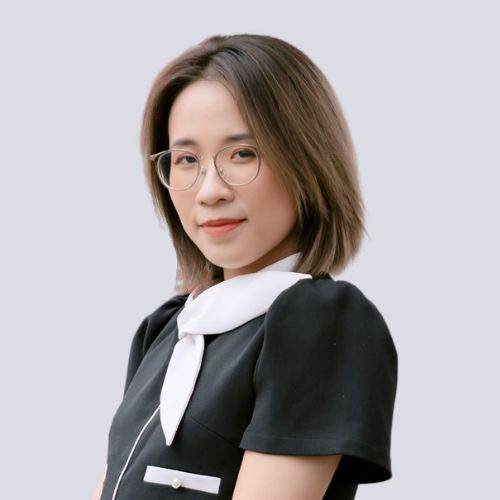
Lucy Pham
Marketing Manager
Comments (1)
-
Solving material you have given to Google, I must say a genuine content! I am just reaching out because I recently published a content that might be a good fit. Either way Keep up the good work.