Magento 2 allows store owners to sell products in many countries by accepting different currencies which is familiar with local buyers. The Manage Currency Symbols feature gives you the ability to customize the symbol associated with each currency that is accepted as payment in your store.
In this blog, I will show you how to get the currency symbol of store view and transform numbers to pricing.
1. Get currency symbol
Retrieve Currency symbol for the current store or any specific store currency symbol by store id or name.
Use Interface to fetch symbol Magento\Framework\Pricing\PriceCurrencyInterface with getCurrencySymbol() method.
Base Syntax:
/** * @param null|string|bool|int|\Magento\Framework\App\ScopeInterface $scope * @param \Magento\Framework\Model\AbstractModel|string|null $currency * @return string */ public function getCurrencySymbol($scope = null, $currency = null);
To use the function you can create Block or Model Class:
<?php namespace Magenest\Currency\Model; use Magento\Framework\Pricing\PriceCurrencyInterface; class CurrencySymbol { /** * @var PriceCurrencyInterface */ private $priceCurrency; public function __construct( PriceCurrencyInterface $priceCurrency ) { $this->priceCurrency = $priceCurrency; } /** * result * * @return string */ public function getCurrencySymbol() { // DEFAULT STORE $currencySymbol = $this->priceCurrency->getCurrencySymbol(); // USE STORE ID $currencySymbol = $this->priceCurrency->getCurrencySymbol(1); // USE STORE CODE $currencySymbol = $this->priceCurrency->getCurrencySymbol('default'); return $currencySymbol; } }
To use it you need $echo $currencySymbol = $this->getCurrencySymbol(); in the template you want.
You can pass the store id, store code as the first parameter in the method or if you have the empty value, the result will be the default store currency code.
2. Transform number float to pricing (PHP & Javascript)
You can get a formatted price with currency in Magento 2 using the block method. Convert a plain number into a price format with a currency symbol using any one of the solutions given below
Base syntax:
/** * Convert and format price value for current application store * * @param float $value * @param bool $format * @param bool $includeContainer * @return float|string */ public function currency($value, $format = true, $includeContainer = true) { return $format ? $this->priceCurrency->convertAndFormat($value, $includeContainer) : $this->priceCurrency->convert($value); }
To use the function you can create Block or Model Class:
<?php namespace Magenest\Currency\Model; use Magento\Framework\Pricing\Helper\Data; /** * Class CurrencyFormat * @package Magenest\Currency\Model */ class CurrencyFormat { /** * @var Data */ protected $priceHelper; /** * CurrencyFormat constructor. * @param Data $priceHelper */ public function __construct(Data $priceHelper) { $this->priceHelper = $priceHelper; } /** * @param $price * @return float|string */ public function getFormattedPrice($price) { return $this->priceHelper->currency($price, true, false); } }
You can use the function getFormattedPrice($price) to format any number you want.
- The first parameter is number formatted.
- The second parameter is true - agree to the format.
- Third parameter is false - disagree with the returned span tag including price inside.
Besides format price in PHP, Magento 2 also supports format price in javascript
You have to use ‘Magento_Catalog/js/price-utils’ priceUtils Js Object in your javascript file.
- priceUtils.formatPrice(amount, priceFormat, showSign) used to format a price.
- amount = required field
- priceFormat = Optional ObjectshowSign = Optional (Show sign like positive or negative)
define([ 'Magento_Catalog/js/price-utils' ], function ( priceUtils ) { 'use strict'; return { /** * Formats the price according to store * * @param {number} Price to be formatted * @return {string} Returns the formatted price */ getFormattedPrice: function (price) { var priceFormat = { decimalSymbol: '.', groupLength: 3, groupSymbol: ",", integerRequired: false, pattern: "$%s", precision: 2, requiredPrecision: 2 }; return priceUtils.formatPrice(price, priceFormat); } } });
Call function from template or js file. You can change priceFormat object based on your requirement.
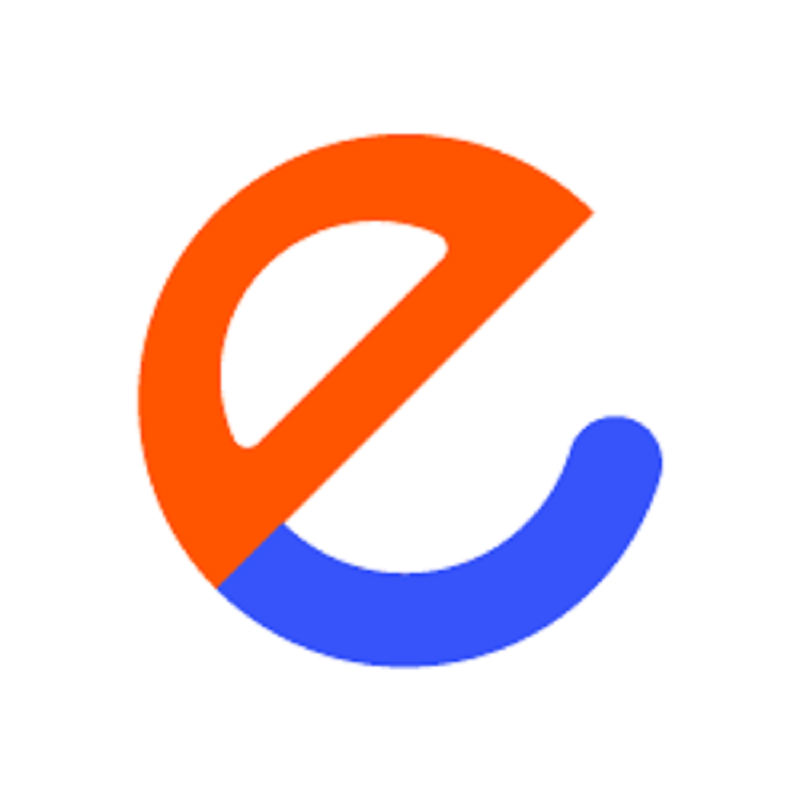