The Magento widgets system is a graphical interface where you can configure blocks in the frontend. For every widget, there is a configuration page available where you can set the required values for that widget. With a Magento widget, you can configure the layout instructions to show the widget at various places in the frontend.
The widget provides powerful features in Magento 2, used to add dynamic or static content to store’s pages and have designed with a set of advanced configuration options.
What we can do with Widget?
- Used to provide information and marketing content via the Magento Administrator panel
- The widget can “call” them from anywhere on the site
- Also allow administrators to add interactive interfaces and features in the Magento front-end
1, Create File widget.xml
The first, We will declare the widget using the widget.xml file in the etc directoryCreate a file app/code/Magenest/CustomWidget/etc/widget.xml with the following content:
<?xml version="1.0" ?> <widgets xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:codetheatres:Magento_Widget:etc/widget.xsd"> <widget class="Magenest\CustomWidget\Block\Widget\ShowProduct" id="magenest_show_product_slider_on_home_page"> <label>Magenest Show Product Slider On Home Page</label> <description>Magenest Show Product On Slider Home Page</description> <parameters> <parameter name="Title" xsi:type="text" required="false" visible="true" sort_order="40"> <label translate="true">Title </label> </parameter> <parameter name="product_id" xsi:type="block" visible="true" required="true" sort_order="30"> <label translate="true">Product</label> <block class="Magento\Catalog\Block\Adminhtml\Product\Widget\Chooser"> <data> <item name="button" xsi:type="array"> <item name="open" xsi:type="string">Select Product...</item> </item> </data> </block> </parameter> </parameters> </widget> </widgets>
With class="Magenest\CustomWidget\Block\Widget\ShowProduct"
having an important, it will handle logic and return the result for frontend.
The element <description>..</description>
and <label>..</label>
show tags for the widget explanation.
In element <parameter...>
have property about:
name
:
show name for field-
xsi:type="text"
:
is parameter type input -
visible
:
is hidden or show fields. -
required
:
Field instance is required. -
sort_order
:
define the position for fields
Widget has parameter types for improved CMS Page building in Magento 2. parameter types like an image selector, WYSIWYG text editor, or textarea.
Here we add some parameter :
- Title: this will show information on the frontend, it element type input
- product_id: is entity_id for a product, this will be selected
In addition, you can customize the params (chooser) for widgets by creating a class and extending the class: Magento\Catalog\Block\Adminhtml\Product\Widget\Chooser
2, Create widget Block class
Second, we create a block class. with this class is responsible for providing data for template, Specifically here "product.phtml"
Create a block file: app/code/Magenest\Magenest_CustomWidget\Block\Widget\ShowProduct.php
<?php /** * Copyright © 2019 Magenest. All rights reserved. * See COPYING.txt for license details. * * Magenest_magento233_dev extension * NOTICE OF LICENSE * * @category Magenest * @package Magenest_magento233_dev */ namespace Magenest\CustomWidget\Block\Widget; use Magento\Catalog\Api\CategoryRepositoryInterface; use Magento\Catalog\Block\Product\Context; use Magento\Catalog\Block\Product\ListProduct; use Magento\Catalog\Model\Layer\Resolver; use Magento\Catalog\Model\ProductFactory; use Magento\Framework\Data\Helper\PostHelper; use Magento\Framework\Url\Helper\Data; use Magento\Widget\Block\BlockInterface; class ShowProduct extends ListProduct implements BlockInterface { protected $_productFactory; public function __construct(Context $context, PostHelper $postDataHelper, Resolver $layerResolver, ProductFactory $productFactory, CategoryRepositoryInterface $categoryRepository, Data $urlHelper, array $data = []) { $this->_productFactory = $productFactory; parent::__construct($context, $postDataHelper, $layerResolver, $categoryRepository, $urlHelper, $data); $this->setTemplate("Magenest_CustomWidget::widget/product.phtml"); } public function getProductInformation(){ $productId = $this->getProduct_id(); if ($productId){ $productId = str_replace('product/','',$productId); } $product = $this->_productFactory->create()->load($productId); return $product; } }
We set template for this block use function: "
$this->setTemplate("Magenest_CustomWidget::widget/product.phtml");
"
With:
Magenest_CustomWidget
:
Name for modulewidget/product.phtml
:
link to view/template
The function "
getProductInformation()
"
is responsible for getting the id_product that we have selected for the product on the admin, it will load the product data with id_product and return the data for some template calls to this function.
3, Create a widget template file in Magento 2
This template will show data for the product use block above.
File: app/code/Magenest/CustomWidget/view/widget/product.phtml
<?php /** * Copyright © 2019 Magenest. All rights reserved. * See COPYING.txt for license details. * * Magenest_magento233_dev extension * NOTICE OF LICENSE * * @category Magenest * @package Magenest_magento233_dev */ /** @var $block Magenest\CustomWidget\Block\Widget\ShowProduct*/ $product = $block->getProductInformation(); $titleWidget = $block->getTitle(); ?> <div> <h2><?=$titleWidget?></h2> <p>This is sample widget show information product.</p> <h3>Product name: <?=$product->getName()?></h3> <h3>Price : <?=$product->getFormattedPrice()?></h3> </div>
4, Flush cache and show widget on Home Page
You should flush Magento cache use command: php bin/magento cache:flush
And We go to admin panel > Content > Pages > Home page > Edit
In Content
tab, click on Insert Widget icon
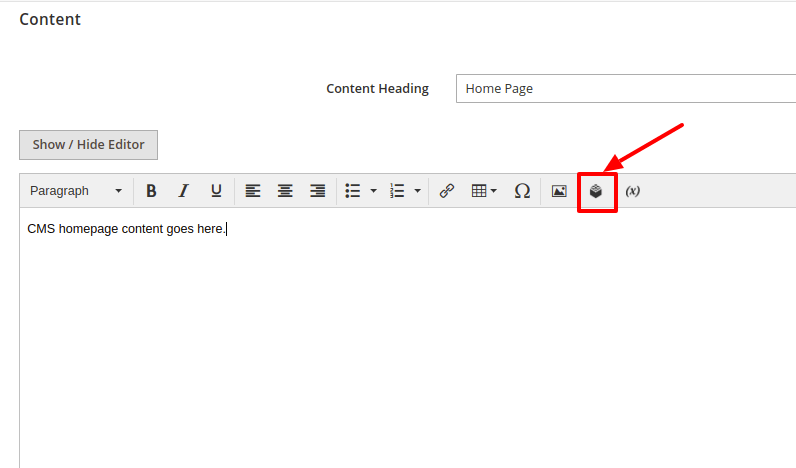
You will see the "Magenest Show Product Slider Widget"
in widget list.
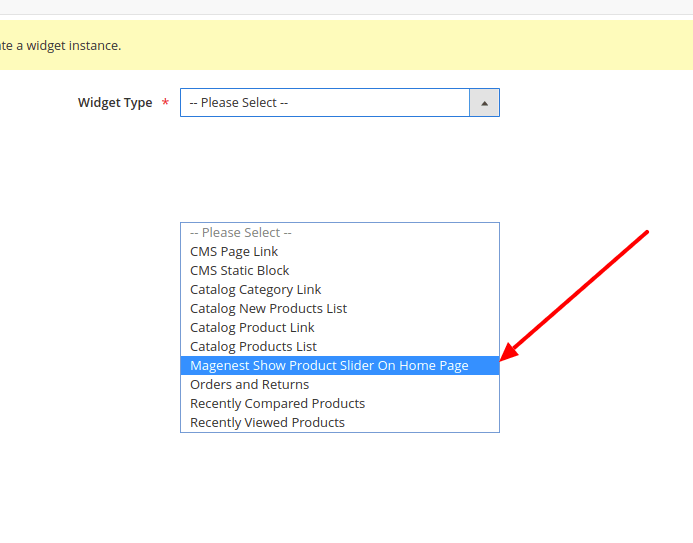
Add content for the widget.
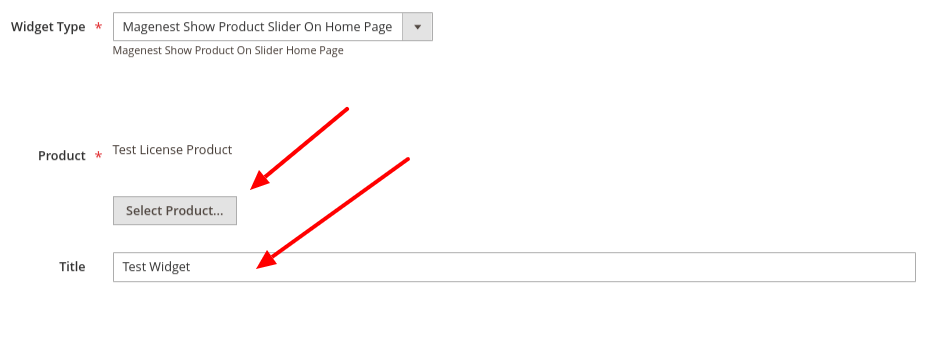
And finally, we have the result on Home Page like this.
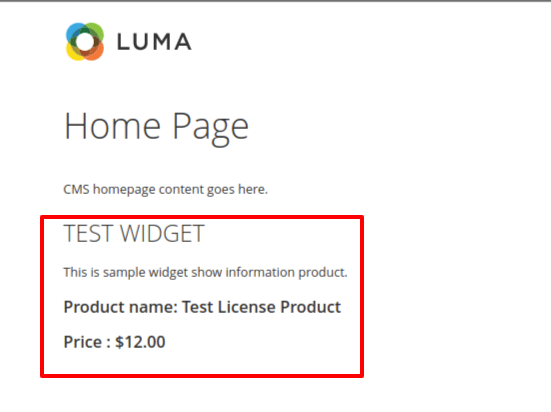
We hope that you can create a custom widget in Magento 2 successfully with these 4 simple steps. Happy coding!
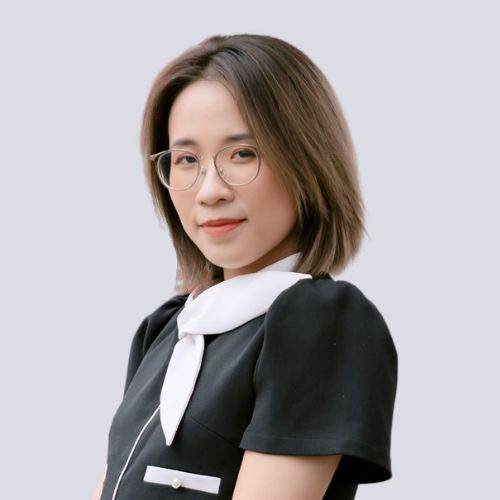