Introduction
In this article, you will be learning how one can send HTTP request in Magento 2.
How to send Magento 2 HTTP request
- First, create an instance of Magento\Framework\HTTP\Client\Curl as below
/** * @var \Magento\Framework\HTTP\Client\Curl */ protected $curl; /** * constructor. * @param \Magento\Framework\HTTP\Client\Curl $curl */ public function __construct( \Magento\Framework\HTTP\Client\Curl $curl ) { $this->curl = $curl; }
- HTTP Header
$this->_curl->addHeader("Content-Type", "application/json"); $this->_curl->addHeader("Content-Length", 200);
The addHeader method accepts two parameters. The request header name and a cURL header value.
- Set Authorization
$userName = "User_Name"; $password = "User_Password"; $this->curl->setCredentials($userName, $password);
It will be equivalent to setting CURLOPT_HTTPHEADER value.
"Authorization : " . "Basic " . base64_encode($userName . ":" . $password)
- Set option
$this->curl->setOption(CURLOPT_RETURNTRANSFER, true); $this->curl->setOption(CURLOPT_PORT, 8080);
The addHeader method accepts two parameters. The request option name and option value.
- Set cookie
$this->curl->addCookie("cookie-name", "cookie-value");
The addCookie method accepts an array as a parameter. The cookie name and the cookie value.
- Get Request
// get method $this->curl->get($url); // output of curl request $result = $this->curl->getBody();
- $url: Contain the endpoint URL.
- $response: It will contain the response of the request.
- Post Request
// post method $this->curl->post($url, $params); // output of curl request $result = $this->curl->getBody();
- $url: Contain the endpoint URL.
- $response: it’s an array, if you want to attach extra parameters in URL.
- $response: It will contain the response of the request.
Example params:
$params = [ 'amount' => 200, 'currency' => 'USD' ]
Example response:
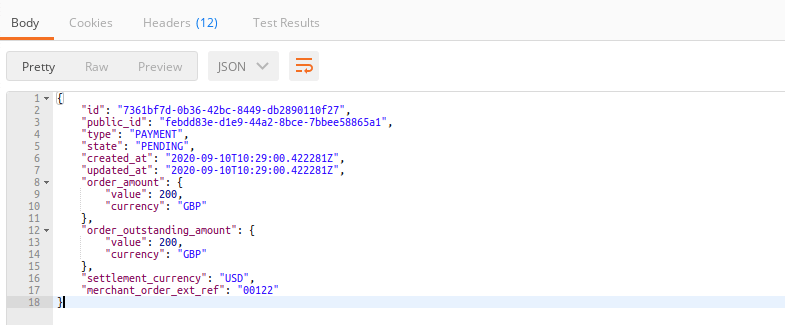
Conclusion
Above is the guideline to send Magento 2 HTTP request. We hope you have learned something useful from this article. If any problem occurs, let us know in the comments. Thank you for reading the article!
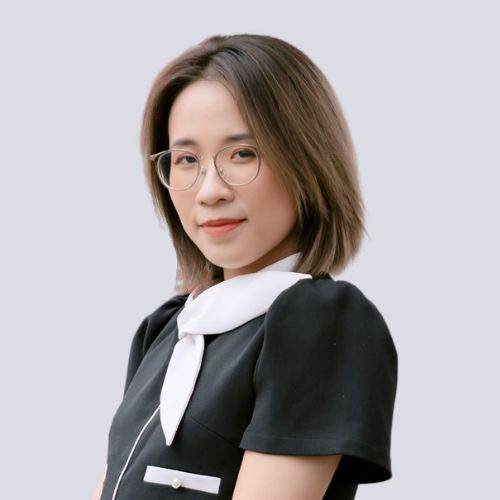