Magento 2 Wishlist allows users to add products to their favorites, which will save time for the next purchases. Also, the wishlists can also be shared with anyone via email.
This article will guide you on how to add a product to the wishlist in Magento 2 programmatically.
How to Add Product to Wishlist
To add a product to a wishlist, we need two parameters: product object and customer id.
- Product Object: can be obtained by Product SKU or Id. For example, use this method to obtain the product from SKU:
Magento\Catalog\Api\ProductRepositoryInterface::get($sku)
- Customer Id. On frontend customer ID can be obtained for the currently logged customer using this method:
Magento\Customer\Model\Session::getId()
To save the product, first load the customer’s wishlist, then add the product via the Wishlist model.
Coding sample:
<?php class AddProductToWishlist { protected $_wishlistFactory; protected $_wishlistResource; public function __construct( Magento\Wishlist\Model\WishlistFactory $wishlistFactory, Magento\Wishlist\Model\ResourceModel\Wishlist $wishlistResource ) { $this->_wishlistFactory = $wishlistFactory; $this->_wishlistResource = $wishlistResource; } /** * @param $product * @param $customerId * * @throws \Magento\Framework\Exception\AlreadyExistsException * @throws \Magento\Framework\Exception\LocalizedException */ public function saveProductToWishlist($product, $customerId) { //load wishlist by customer id $wishlist = $this->_wishlistFactory->create()->loadByCustomerId($customerId, true); //add product for wishlist $wishlist->addNewItem($product); //save wishlist $this->_wishlistResource->save($wishlist); } }
And here is the result: The product has been added to the user's favorite list successfully in the frontend.
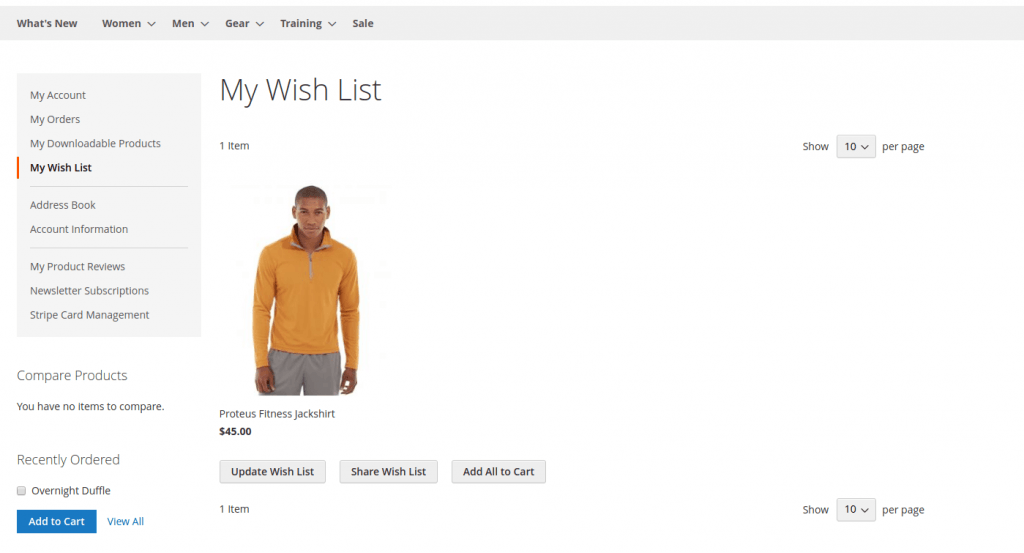
Conclusion
Magento 2 Wishlist is a convenient way to keep track of products that you like, but are not ready to buy. Items from your wish list can be shared with others or added to the shopping cart. It brings a better experience for users.
By default, Magento supports only one wishlist for each customer. For Multiple Wishlists, you can check out our free extension here.
We hope you have learned something useful from this article. If any problem occurs, let us know in the comments.
Thank you for reading!
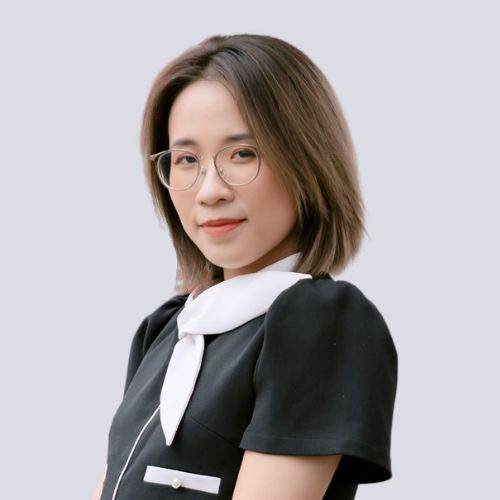