Introduction
Have you ever encountered troubles while trying to create a simple product in Magento 2? If you have, then we have something for you today. This tech blog, Magenest will guide you to programmatically create a simple product in Magento 2. Not only so, but we will also carefully explain the sample code we give out to you guys too.
Without keeping you guys waiting anymore, let's take a look
Create a simple product programmatically
Sample Code
<?php namespace /** * @var \Magento\Catalog\Model\ProductFactory */ protected $_productFactory; /** * @var \Magento\Catalog\Model\ResourceModel\Product */ protected $_resourceModel; /** * @var SourceItemInterface */ protected $sourceItemsSaveInterface; /** * @var \Magento\InventoryApi\Api\Data\SourceItemInterfaceFactory */ protected $sourceItem; /** * @var \Magento\Eav\Api\AttributeRepositoryInterface */ protected $attributeRepository; /** * @var \Magento\ConfigurableProduct\Helper\Product\Options\Factory */ protected $_optionsFactory; /** * @var \Magento\Catalog\Api\ProductRepositoryInterface */ protected $productRepository; /** * @var \Magento\Catalog\Setup\CategorySetup */ protected $categorySetup; /** * @var \Magento\Store\Model\StoreManagerInterface */ protected $storeManager; public function __construct( \Magento\Catalog\Model\ProductFactory $productFactory, \Magento\Catalog\Model\ResourceModel\Product $resourceModel, \Magento\InventoryApi\Api\Data\SourceItemInterface $sourceItemsSaveInterface, \Magento\InventoryApi\Api\Data\SourceItemInterfaceFactory $sourceItem, \Magento\Eav\Api\AttributeRepositoryInterface $attributeRepository, \Magento\ConfigurableProduct\Helper\Product\Options\Factory $optionsFactory, \Magento\Catalog\Api\ProductRepositoryInterface $productRepository, \Magento\Catalog\Setup\CategorySetup $categorySetup, \Magento\Store\Model\StoreManagerInterface $storeManager, Context $context ) { parent::__construct($context); $this->_productFactory = $productFactory; $this->_resourceModel = $resourceModel; $this->sourceItemsSaveInterface = $sourceItemsSaveInterface; $this->sourceItem = $sourceItem; $this->attributeRepository = $attributeRepository; $this->_optionsFactory = $optionsFactory; $this->productRepository = $productRepository; $this->categorySetup = $categorySetup; $this->storeManager = $storeManager; } public function execute() { $product = $this->_productFactory->create(); $colorAttr = $this->attributeRepository->get(Product::ENTITY, 'color'); $bottomSetId = $this->categorySetup->getAttributeSetId(Product::ENTITY, 'Bottom'); $associatedProductIds = []; $options = $colorAttr->getOptions(); $values = []; $sourceItems = []; array_shift($options); try { //Create Simple product foreach ($options as $index => $option) { $product->unsetData(); $product->setTypeId(Type::TYPE_SIMPLE) ->setAttributeSetId($bottomSetId) ->setWebsiteIds([[$this->storeManager->getDefaultStoreView()->getWebsiteId()]]) ->setName('Sample-' . $option->getLabel()) ->setSku('simple_' . $index) ->setPrice(100) ->setVisibility(Visibility::VISIBILITY_BOTH) ->setStatus(Status::STATUS_ENABLED) // Assign category for product ->setCategoryIds([2]); // Category Default $product->setCustomAttribute( $colorAttr->getAttributeCode(), $option->getValue() ); $this->_resourceModel->save($product); // Update Stock Data $sourceItem = $this->sourceItem->create(); $sourceItem->setSourceCode('default'); $sourceItem->setQuantity(100); $sourceItem->setSku('simple_' . $index); $sourceItem->setStatus(SourceItemInterface::STATUS_IN_STOCK); } //Execute Update Stock Data $this->sourceItemsSaveInterface->execute($sourceItems); } catch (\Exception $e) { echo $e->getMessage(); } }
Sample code explanation
- $this->productFactory->create() creates the product object. Once the object is created, properties of the product such as SKU, name, and description will be set.
- $product->setWebsiteIds([$this->storeManager->getDefaultStoreView()->getWebsiteId()]) assigns the product to one website and if there are multiple websites for which the same product is to be assigned, then you could call the website IDs through the store.
- $product->setAttributeSetId($bottomSetId): set Attribute for product. In our example $bottomSetId = $this->categorySetup->getAttributeSetId(Product::ENTITY, 'Bottom'). You can set another Attribute Set for a simple product.
- $product->setAttributeSetId($bottomSetId): set Attribute for product. In our example $bottomSetId = $this->categorySetup->getAttributeSetId(Product::ENTITY, 'Bottom'). You can set another Attribute Set for a simple product.
- $product->setTypeId(\Magento\Catalog\Model\Product\Type::TYPE_SIMPLE) sets product as a simple product.
- $product->setVisibility(\Magento\Catalog\Model\Product\Visibility::VISIBILITY_BOTH) sets product visibility as both catalog and search. VISIBILITY_IN_CATALOG sets product visibility as a catalog, VISIBILITY_IN_SEARCH sets product visibility as search, and VISIBILITY_NOT_VISIBLE makes the product not visible individually.
- $product->setStatus(\Magento\Catalog\Model\Product\Attribute\Source\Status::STATUS_ENABLED) makes the product enabled and “STATUS_DISABLED” makes the product disabled.
- In Magento 2.3 was deprecated change stock directly via setStockData(). So, to update Stock Data for product with Magento MSI, please add this to the constructor:
public function __construct( \Magento\InventoryApi\Api\Data\SourceItemInterface $sourceItemsSaveInterface, \Magento\InventoryApi\Api\Data\SourceItemInterfaceFactory $sourceItem, ) { $this->sourceItemsSaveInterface = $sourceItemsSaveInterface; $this->sourceItem = $sourceItem;
- $sourceItem->setSourceCode('default'): Using the “default” inventory source, which is added to Magento by the install script and it’s there unless it was removed from the admin
- $sourceItem->setQuantity(100): Set quantity for product
- $sourceItem->setSku('simple_' . $index): the product SKU
- $sourceItem->setStatus(SourceItemInterface::STATUS_IN_STOCK): Set Stock Status for product ( STATUS_IN_STOCK or STATUS_OUT_OF_STOCK)
- $this->sourceItemsSaveInterface->execute($sourceItems): SourceItemsSaveInterface accepts an array of items and it’s quite fast to save multiple stock items at once, compared to saving them one by one in a loop.
The static details can be set based on the requirements. If you are looking for creating multiple products programmatically by the script at that time, you can use this script and customize code for multiple products created.
Conclusions
In this blog, we have covered the basics of creating a simple product in Magento 2. Check other tech guides from Magenest right here for detailed guidelines, instructions, and sample codes for Magento 2 technical issues.
If you have any questions, feel free to leave your comments below or send us a message via our support line.
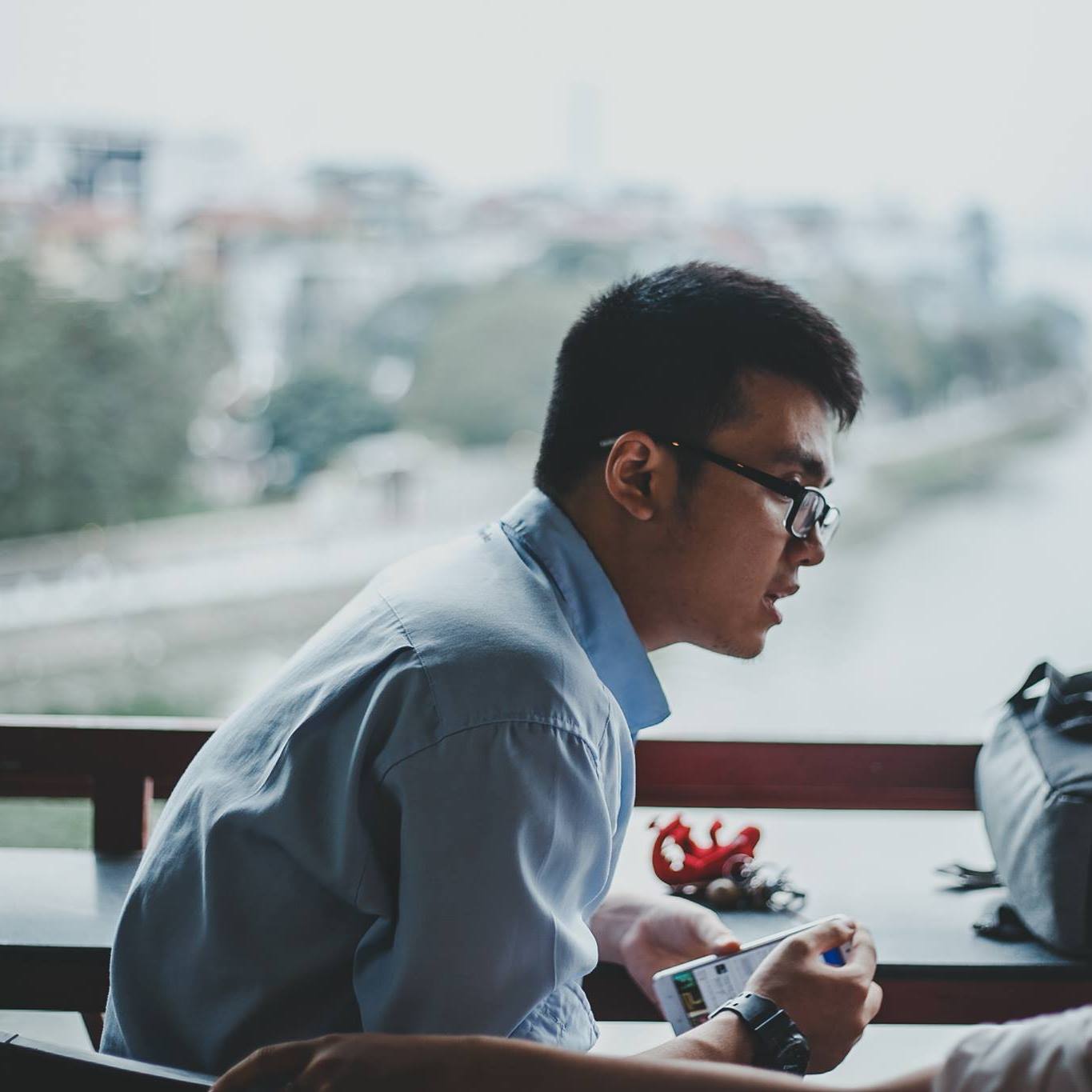