Understanding customers is the key to the success of all eCommerce businesses. In Magento 2, merchants can collect customer attributes through various fields in the registration form, account dashboard, etc. To have better insights into your customers, adding custom attributes to the customer grid is necessary. In this blog, we'd like to guide you on how to create custom customer attributes in Magento 2 with 2 simple steps.
Smart solution for you: Use Customer Attributes for Magento 2 to collect customer information effectively.
Step 1: Create a data patch
The latest version of Magento introduces a new way to modify data in Magento 2 database: Data / Schema Patches (further info here: Magento Documentation).
We will implement a data patch to add a customer’s attribute to the database.
First, create a class named: CustomerAttributePatcher
in your module directory following this directory convention: <your-module>/Setup/Patch/Data/CustomerAttributePatcher.php
Note: All data patches must be in this directory.
Must implement Magento\Framework\Setup\Patch\DataPatchInterface
<?php namespace Magenest\Customer\Setup\Patch\Data; use Magento\Customer\Model\Customer; use Magento\Eav\Model\Config; use Magento\Customer\Setup\CustomerSetupFactory; use Magento\Framework\Setup\ModuleDataSetupInterface; use Magento\Framework\Setup\Patch\DataPatchInterface; class AccountPurposeCustomerAttribute implements DataPatchInterface { /** * @var CustomerSetupFactory */ private $customerSetupFactory; /** * @var ModuleDataSetupInterface */ private $setup; /** * @var Config */ private $eavConfig; /** * AccountPurposeCustomerAttribute constructor. * @param ModuleDataSetupInterface $setup * @param Config $eavConfig * @param CustomerSetupFactory $customerSetupFactory */ public function __construct( ModuleDataSetupInterface $setup, Config $eavConfig, CustomerSetupFactory $customerSetupFactory ) { $this->customerSetupFactory = $customerSetupFactory; $this->setup = $setup; $this->eavConfig = $eavConfig; } /** We'll add our customer attribute here */ public function apply() { $customerSetup = $this->customerSetupFactory->create(['setup' => $this->setup]); $customerEntity = $customerSetup->getEavConfig()->getEntityType(Customer::ENTITY); $attributeSetId = $customerSetup->getDefaultAttributeSetId($customerEntity->getEntityTypeId()); $attributeGroup = $customerSetup->getDefaultAttributeGroupId($customerEntity->getEntityTypeId(), $attributeSetId); $customerSetup->addAttribute(Customer::ENTITY, 'new_attribute', [ 'type' => 'int', 'input' => 'select', 'label' => 'New Attribute', 'required' => true, 'default' => 0, 'visible' => true, 'user_defined' => true, 'system' => false, 'is_visible_in_grid' => true, 'is_used_in_grid' => true, 'is_filterable_in_grid' => true, 'is_searchable_in_grid' => true, 'position' => 300 ]); $newAttribute = $this->eavConfig->getAttribute(Customer::ENTITY, 'new_attribute'); $newAttribute->addData([ 'used_in_forms' => ['adminhtml_checkout','adminhtml_customer','customer_account_edit','customer_account_create'], 'attribute_set_id' => $attributeSetId, 'attribute_group_id' => $attributeGroup ]); $newAttribute->save(); } public static function getDependencies() { return []; } public function getAliases() { return []; } }
Step 2: Install the data patch
Run upgrade command in order for the data patch to take action: php bin/magento setup:upgrade
Now our customer attribute is installed.
We hope these simple steps can assist you in adding the custom customer attribute in Magento 2 you need. If you have any questions, please put them in the comment.
READ MORE. How to check if a customer is logged in Magento 2?
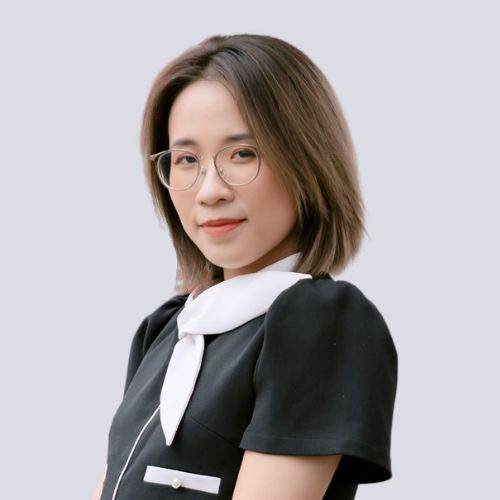