Introduction
Cache helps to increase the page load speed by storing the web pages through browsers. Also, it reduces resource requirements in the situation of heavy traffic. Everyone wants the fastest way to clean and flush cache in Magento 2! This blog will show you how to clean, flush cache in Magento 2 programmatically.
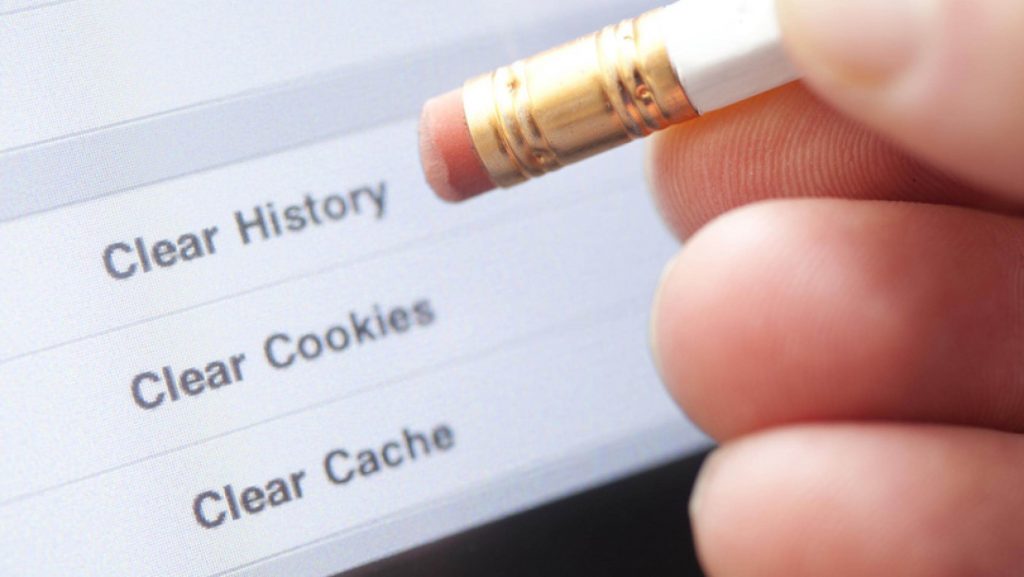
Clean and flush cache by cache types in Magento 2 programmatically
There are 4 ways you can clean cache by cache types in Magento 2 programmatically:
The first way:
- Pass the argument to the constructor
Magento\Framework\App\Cache\TypeListInterface $typeList of a required class (Repository, Model, Block, etc)
Sample code:
/** * @param TypeListInterface $typeList */ public function __construct(TypeListInterface $typeList) { $this->typeList = $typeList; }
- To clean cache we use the function cleanType() in TypeListInterface
This step will clean cached data for specific cache types. Now add following code to the method where you want clear cache
Sample code:
/* get all types of cache in system */ $allTypes = array_keys($this->typeList->getTypes()); foreach ($allTypes as $type) { $this->typeList->cleanType($type); }
$allTypes will return the array of all cache type, you can also declare the cache type that you want to clean such as:
$allType = ['config','layout','block_html','collections','reflection','db_ddl', 'eav', 'customer_notification','config_integration','config_integration_api','full_page', 'translate', 'config_webservice']
The second way
Sample code:
public function __construct( \Magento\Framework\App\Cache\Manager $cacheManager ) { $this->cacheManager = $cacheManager; } private function whereYouNeedToCleanCache() { $this->cacheManager->clean($this->cacheManager->getAvailableTypes()); }
Now, let’s understand the purpose of codes implemented:
+ $this->cacheManager->getAvailableTypes(): It will return the array of cache type
+ $this->cacheManager->clean(): Cleans up caches
The third way
- Define constructor – pass
Magento\Framework\App\Cache\Frontend\Pool to your constructor
Sample code:
public function __construct( \Magento\Framework\App\Cache\Frontend\Pool $cacheFrontendPool ) $this->cacheFrontendPool = $cacheFrontendPool; }
- Now add the following code to the method where you want to flush cache
/* Flushed the Entire cache storage from system, Works like Flush Cache Storage button click on System -> Cache Management */ foreach ($this->cacheFrontendPool as $cacheFrontend) { $cacheFrontend->getBackend()->clean(); }
The fourth way
Sample code:
public function __construct( \Magento\Framework\App\Cache\Manager $cacheManager ) { $this->cacheManager = $cacheManager; } private function whereYouNeedToFlushCache() { $this->cacheManager->flush($this->cacheManager->getAvailableTypes()); }
Clear cache by cache tags in Magento2 programmatically
Magento 2 uses Cache Tag as an identifier for each block of code. It renders HTML output, allows the Magento Full page cache to check, and invalidates the date (private-content invalidating will be handled at the client-side).
$this->_eventManager->dispatch('clean_cache_by_tags', ['object' => $cache]);
The cache tags are created at the block level. Each block class implements the Identity Interface. It means they must implement a getIdentities method, which must return a unique identifier.
Sample code:
... namespace Magento\Cms\Block; ... use Magento\Framework\View\Element\AbstractBlock; use Magento\Framework\DataObject\IdentityInterface; ... class Page extends AbstractBlock implements IdentityInterface { public function getIdentities() { return [\Magento\Cms\Model\Page::CACHE_TAG . '_' . $this->getPage()->getId()]; } }
Clear cache by cache id in Magento2 programmatically
Sample code:
<?php namespace YourVendor\YourModule\Model; use Magento\Framework\App\ObjectManage; use Magento\Framework\App\CacheInterface; class YourmoduleStorage { /** * Cache id * * @var string */ const CACHE_ID = 'yourmodule_custom_cache'; /** * Cache tag * * @var string */ const CACHE_TAG = 'data'; /** * * @var CacheInterface */ private $cache; public function __construct(CacheInterface $cache) { $this->cache = $cache; } /** * Remove cache * * @param string $cacheId * @param string $data */ public function remove($cacheId, $data) { $this->cache->remove($this->getCacheKey($cacheId, $data)); } /** * Get Cache key * * @param string $cacheId * @param string $data * @return string */ private function getCacheKey($cacheId, $data) { return 'yourmodule_cache_type_' . $cacheId . '_' . $data; } }
Now, let’s understand the purpose of the codes implemented. We use the function remove() in CacheInterface. It will remove cached data by identifier.
Conclusion
In this blog, we’ve shown you simple ways to clean, flush cache in Magento 2 programmatically. We hope that this blog has answered the questions you have along the process of cleaning and flushing cache. If you have any other issues, feel free to leave your comments below.
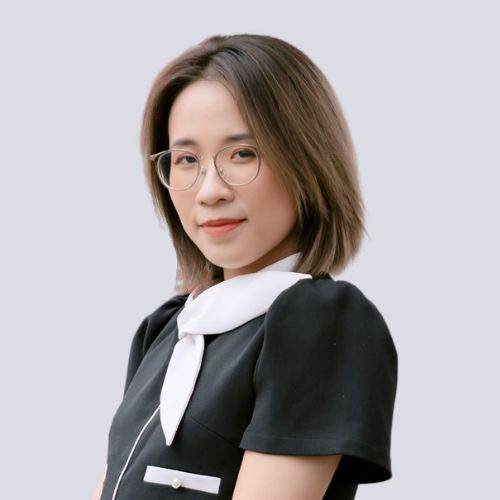