Cookies are a necessary part of the way the web works. Websites use cookies to keep track of users and enable user-specific features. So in this article, we will show you guys how to get and set the data from cookies in Magento 2.
Create Class to manager cookie
The first step is setting an ExampleCookie.php class in the app/code/Magenest/Helper. The ExampleCookie.php contains the following content:
<?php namespace Magenest\ManagerCookie\Helper; use Magento\Framework\Session\SessionManagerInterface; class ExampleCookie { protected $cookieManager; protected $cookieMetadataFactory; public function __construct( \Magento\Framework\Stdlib\CookieManagerInterface $cookieManager, \Magento\Framework\Stdlib\Cookie\CookieMetadataFactory $cookieMetadataFactory ) { $this->cookieManager = $cookieManager; $this->cookieMetadataFactory = $cookieMetadataFactory; } /** * get cookie by name */ public function getCookie($cookieName) { return $this->cookieManager->getCookie($cookieName); } /** * set public cookie */ public function setPublicCookie($cookieName, $value) { $metadata = $this->cookieMetadataFactory ->createPublicCookieMetadata() ->setDuration(86400) // Cookie will expire after one day (86400 seconds) ->setSecure(true) //the cookie is only available under HTTPS ->setPath('/subfolder')// The cookie will be available to all pages and subdirectories within the /subfolder path ->setHttpOnly(false); // cookies can be accessed by JS $this->_cookieManager->setPublicCookie( $cookieName, $value, $metadata ); } /** * set sensitive cookie */ public function set Sensitive Cookie($cookieName, $value) { $metadata = $this->cookieMetadataFactory ->createSensitiveCookieMetadata() ->setPath('')// for global ->setDomain('.example.com');// cookie will be available for www.example.com, example.com and will not be available for anotherexample.com $this->_cookieManager->setSensitiveCookie( $cookieName, $value, $metadata ); } }
About setting cookies in Magento, first, you guys need to remember that we have two types of cookies in Magento. They are Public and Sensitive cookie:
- Public cookies can be accessed by JS. HttpOnly will be set to false by default for these cookies.
- Sensitive cookies cannot be accessed by JS on the client-side it just can access on your server. HttpOnly will always be set to true for these cookies.
When you set a cookie, you set the raw cookie data and some metadata. This metadata includes the path for where the cookie is valid, the Duration for expiration time of the cookie, and so on.
Use cookie in Javascript
In javascript you just need to include jquery/jquery.cookie to use cookie
<script type="text/javascript"> require([ 'jquery', 'jquery/jquery.cookie' ], function ($) { $(document).ready(function () { var simple_cookie = $.cookie('name_cookie'); // Get Cookie Value, note: You can only get a cookie if it is public if(!simple_cookie) { $.cookie('name_cookie', 'value of cookie', {expires: 86400}); // Set Cookie with metadata (note the cookies set here are public) }else { console.log(simple_cookie); } }); </script>
Conclusion
It's not too hard to get and set the cookies of Magento 2. However, this process is still a bit tricky for eCommerce newbies. We hope that this article has already contained the answers to your problems.
If it doesn't, then please don't hesitate to drop us an email at support@magenest.com.
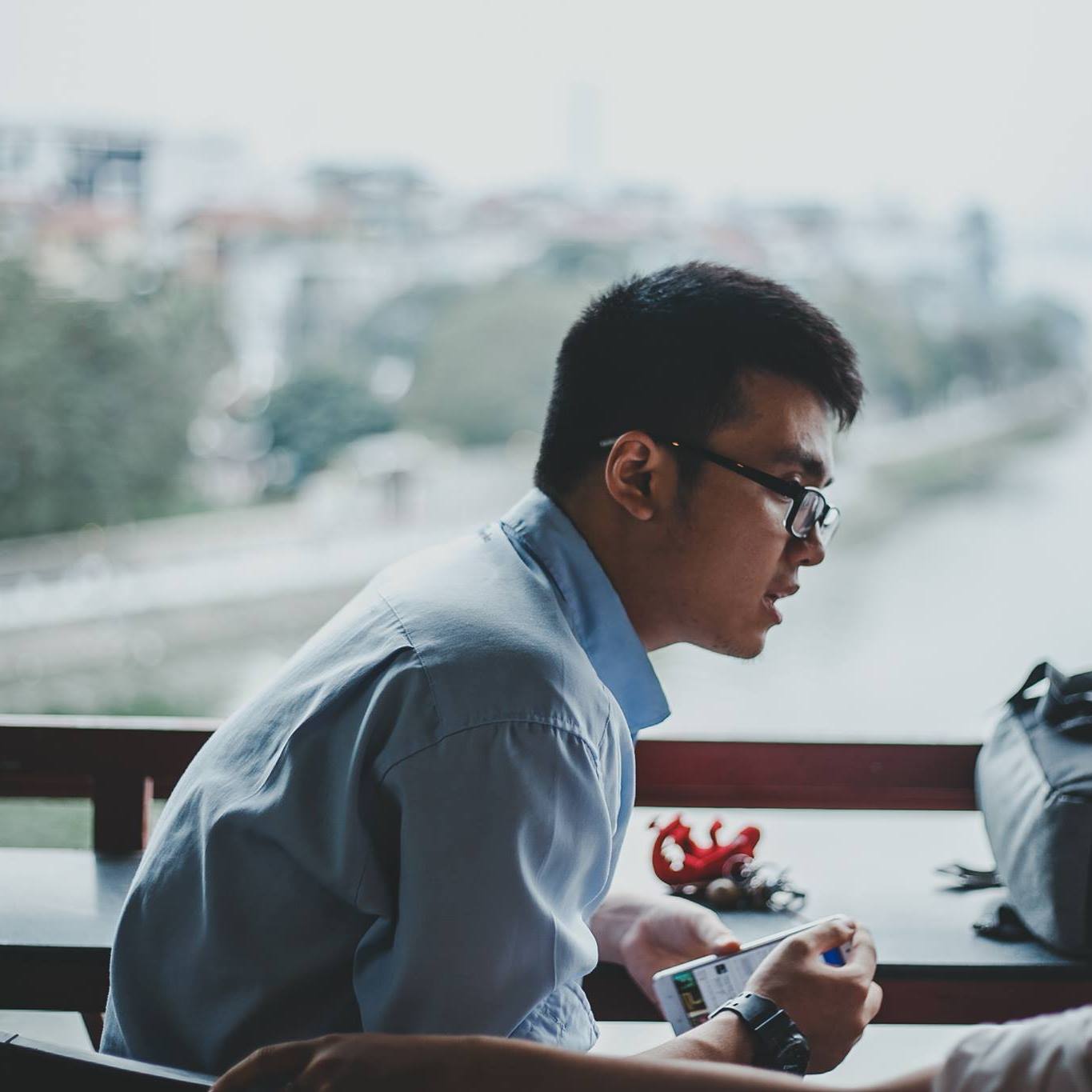